Laravel forgot password custom validation
Laravel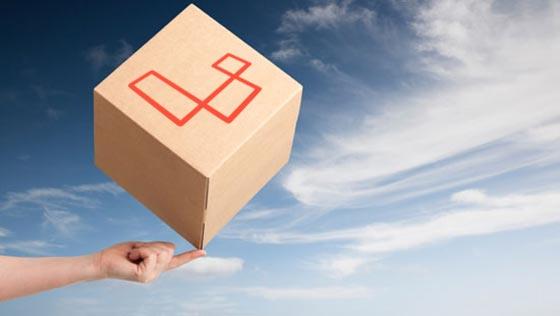
DOES NOT WORK IN L5.4
Copy the function sendResetLinkEmail() from illuminate\Foundation\Auth\SendsPasswordResetEmails.php and place it in your ForgotPasswordController.php this will override the default function.
Make sure to include these under your namespace:
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Password;
/** * Send a reset link to the given user. * * @param \Illuminate\Http\Request $request * @return \Illuminate\Http\RedirectResponse */ public function sendResetLinkEmail(Request $request) { $this->validate($request, ['email' => 'required|email', 'g-recaptcha-response' => 'required|captcha']); // We will send the password reset link to this user. Once we have attempted // to send the link, we will examine the response then see the message we // need to show to the user. Finally, we'll send out a proper response. $response = $this->broker()->sendResetLink( $request->only('email') ); if ($response === Password::RESET_LINK_SENT) { return back()->with('status', trans($response)); } // If an error was returned by the password broker, we will get this message // translated so we can notify a user of the problem. We'll redirect back // to where the users came from so they can attempt this process again. return back()->withErrors( ['email' => trans($response)] ); }