Laravel Mail Queue
Laravel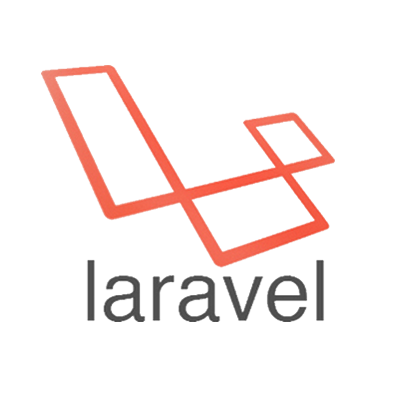
Pulled from http://laravelcoding.com/blog/laravel-5-beauty-sending-mail-and-using-queues
Changing the Controller
To queue the email, there’s only a single small change to make. Update your ContractController
class as instructed.
Change to ContractController.php
// Find the line below
Mail::send('emails.contact', $data, function ($message) use ($data) {
// And change it to match what's below
Mail::queue('emails.contact', $data, function ($message) use ($data) {
That’s it! Instead of Mail::send()
we call Mail::queue()
and Laravel will automatically queue it for us.
Where’s the Email
Test out the Contact Us form again. After you click [Send] there should be no delay before you see the Success message.
Still, you can wait forever for the email and it will never arrive.
Why?
Because there’s no process running in the background, watching for and handling items arriving in the queue.
Running queue:work
To process the next item on the queue, we can manually run artisan’s queue:work
command.
This command will do nothing if the queue is empty. But if there’s an item on the queue it will fetch the item and attempt to execute it.
Running Artisan queue:work
vagrant@homestead:~/Code/l5beauty$ php artisan queue:work
Processed: mailer@handleQueuedMessage
As you can see here it handled the queued email message. Now the email should arrive in your inbox within moments.
Automatically Processing the Queue
Of course, having to manually log into our server and run the artisan queue:work
each time we want to process the next item on the queue is ridiculous.
There’s a few options to automate this.
Using a Scheduled Command
Another option for low volume sites is to schedule queue:work
to run every minute. Or even every 5 minutes. This is best done using Laravel 5.1’s command scheduler.
Edit app/Console/Kernel.php
and make the changes below.
Editing Console Kernel
// Replace the following method
/**
* Define the application's command schedule.
*
* @param Schedule $schedule
* @return void
*/
protected function schedule(Schedule $schedule)
{
// Run once a minute
$schedule->command('queue:work')->cron('* * * * * *');
}
This will run the queue:work
command once a minute. You can change this frequency in many ways.
Various Run Frequencies in Console Kernel
// Run every 5 minutes
$schedule->command('queue:work')->everyFiveMinutes();
// Run once a day
$schedule->command('queue:work')->daily();
// Run Mondays at 8:15am
$schedule->command('queue:work')->weeklyOn(1, '8:15');
To see more options view the documentation.
The second step in setting up the scheduled command is to modify your machine’s crontab. Edit crontab and add the following line.
Crontab Line for Artisan Scheduler
* * * * * php /path/to/artisan schedule:run 1>> /dev/null 2>&1
This will call artisan to run anything currently scheduled, sending any output to the null device.